Write a program that reads in a text file and outputs every second line. The program should take the filename from an argument on the command line.
# Create a text file with line numbers so that I can test my script
with open("my_testfile.txt","w") as f:
for i in range(200):
# add a line number starting at 0 to 200
# convert i to a string to avoid 'TypeError: write() argument must be str, not int'
f.write(str(i))
f.write(" This is line number ")
f.write(str(i))
f.write(" in my new text file \n")
import sys
if len(sys.argv) < 2:
file_to_read = input("please enter a valid .txt file to continue ")
# if the user has entered more than 2 arguments along with the program name alert them:
elif len(sys.argv) > 2:
print(f"You have entered {len(sys.argv)} arguments. Please try again with a single .txt file")
file_to_read = input("To continue please enter a single valid .txt file ")
else:
file_to_read = sys.argv[1]
print(f"the file to read is {file_to_read}")
# after the above block of code, there should be a file_to_read ready for processing.
# using try except statements to print a proper message if the file input is not a valid file.
try:
with open(file_to_read,"r") as f:
line_no = 0
# for every line l in the file object f, keep track of the line numbers and if the line number is even print the line
for l in f:
line_no += 1
if (line_no % 2) ==0:
print(l)
# print exception error if the file is not valid
except FileNotFoundError:
print("That is not a valid file. The program cannot continue")
To run this program, go to the command line and enter the following command: python solution-9.py <file.txt>
. If the text file is not in the same directory as solution-9.py then you should provide the full path to the file.
If the user has not supplied a filename to the program on the command line then they are prompted to enter a file. Check if there is an argument entered at the command line. This will be index 1. The program name is an index 0.
If there is only 1 sys
argument, then the user has not entered a file name.
The program needs exactly one .txt
file so the length of sys.argvs
should be exactly 2.
To test this I downloaded the text file version of Moby Dick from the Gutenberg project at http://www.gutenberg.org/ebooks/2489 (Project Gutenberg offers thousands of free eBooks in various formats - it was the first provider of ebooks) I also created a shorter text file with line numbers so I could actually tell if every second line is printing. If the file entered was invalid, a try - exception was used to alert the user that the file was invalid.
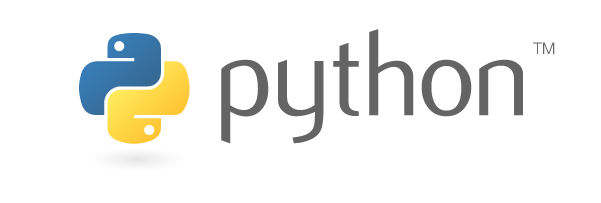