Write a program that outputs today’s date and time in the format “Monday, January 10th 2019 at 1:15pm”.
import datetime as dt
# using the datetime class of the datetime module, call the class method datetime.now()
now = dt.datetime.now()
day = now.strftime("%d")
# Add a suffix such as 'th' for 5th, 'st' for 1st, 'nd' for 2nd etc to get the format shown in the sample
# set up a variable `suf` for this suffix. Let the default suffix be "th" as this is correct for most days of the month
suf = "th"
##First use if statement to check if the day is the 1st, 21st or 31st of the month, if so replace `th` with `st`
if int(day) in (1,21,31):
suf = "st"
# use elif statement to check if the day is 2nd or 22nd, if so replace `th` with `nd`
elif int(day) in (2,22):
suf ="nd"
elif int(day) == 23:
suf ="rd"
# Splitting the date and time into two parts, adding the suf variable in between by concatenating using the + operator, make lowercase
print(now.strftime("%A, %B %d")+suf, now.strftime("%Y at %I:%M%p").lower())
The program takes the current time and date on your computer at the time the program is run. It creates a string containing time and date elements in the required format and prints it. The required format is: A weekday name, followed by a comma “,” then full version of month name followed by the day of month with a suffix for ‘th’ or ‘nd’ or ‘st’ Then the 4 digit year, followed by the string “at” followed by 24 hour digital clock time with “am” or “pm” at the very end. followed by year %y followed by “at” then time using 12 hour clock %I and %p for the suffix of am or pm
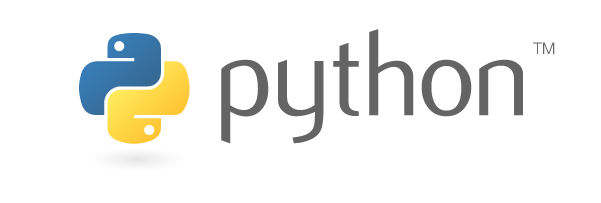