Write a program that asks the user to input a positive integer and tells the user whether or not the number is a prime.
while True:
try:
x = int(input("Please enter a positive integer: "))
if x < 0:
raise ValueError
break
except ValueError:
print("That was not a valid positive integer. Please try again!")
if x == 2:
print(x, "That is a prime")
else:
for n in range(2,x):
if x % n == 0:
print("That is not prime")
break
else:
print("That is prime")
The program will ask the user to input a postive integer. The program will output a message saying if the number is prime or not.
A prime number is a whole number greater than 1 that is only divisible by itself and 1, that is it’s only factors are 1 and the number itself.
To determine if a number greater than 1 is a prime number check if it can be divided, without a remainder, by any number between 2 and the number itself. Once you find any number at all, that divides into it without a remainder then it is not a prime number.
To verify this program was working ok I checked against a selection of prime numbers at Wikipedia.org.
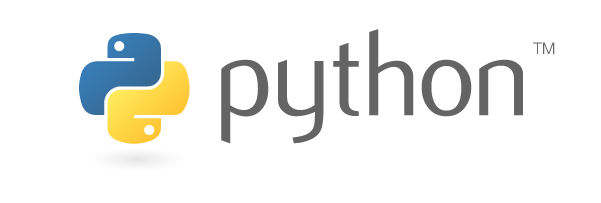