Write a program that asks the user to input any positive integer and outputs the successive values of the following calculation. At each step calculate the next value by taking the current value and, if it is even, divide it by two, but if it is odd, multiply it by three and add one. Have the program end if the current value is one.
while True:
try:
x = int(input("Please enter a positive integer: "))
if x < 0:
raise ValueError
break
except ValueError:
print("That was not a positive integer. Please try again!")
my_seq =[x]
while x > 1:
if x % 2 == 0:
x = x //2
my_seq.append(x)
else:
x = (x * 3) + 1
my_seq.append(x)
print(*my_seq, sep = ", ")
The input must be a positive integer. If the user inputs something other than this an error message is printed until a positive integer is entered using try and except statements. A ValueError
is raised to capture a negative integer being input.
This program uses a while
loop and if-else
statements to check if the previous number in the sequence is even or odd. To produce output in the same format as the sample output provided I used a list to hold the terms of the sequence. The next term in the sequence is appended to the end of the list.
To remove the brackets from the list I used *
to unpack the list and to print the sequence without brackets.
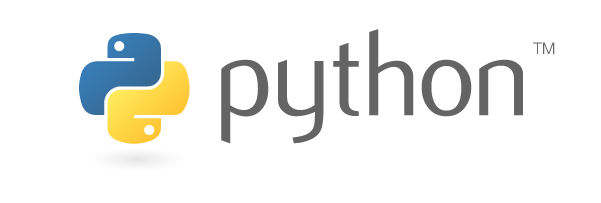