Write a program that prints all numbers between 1,000 and 10,000 that are divisible by 6 but not 12.
A for
loop is used to iterate through every number in a sequence of numbers in the order they appear in the sequence. The range()
function generates a sequence of numbers that can be iterated over in the for loop.
The program will print all the numbers between 1,000 and 10,000 that are divisible by 6 but are not divisible by 12. Any numbers between 1,000 and 10,000 that are divisible by both 6 and 12 are not printed.
for i in range(1000,10000):
if (i % 6 ==0):
if(i % 12 !=0):
print(i)
This program goes through all of the integers between 1000 and 10000. For each number it first checks if it is divisible by 6 and if it is, then it checks if the number is not divisible by 12. If the number is divisible by 6 and is also not divisible by 12 then this number is printed. However if the number is divisible by 6 and is also divisible by 12 then this number is not printed.
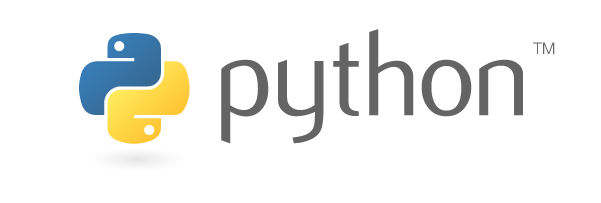