Write a program that outputs whether or not today is a day that begins with the letter T.
This program takes as input the actual day of the week when the program is run. The datetime
module, part of the Python Standard Library, can do this. The date
object of datetime
is used here and it’s class method date.today()
is used to get today’s date - the date when the program is actually run. The program requires only the first letter of the day returned from the date. It extracts a substring containing the first element of the day name. The date object has a method strftime
that will return a string representing the date according to a format string that is explicitly specified date.strftime(format)
. The program then checks if the first letter is equal to “T” or not and prints the appropriate message.
"""
# This program outputs whether or not today is a day
that begins with the letter T.
"""
from datetime import date
if date.today().strftime("%A")[0] == "T":
print("Yes - today begins with a T")
else:
print("No - today does not begin with a T")
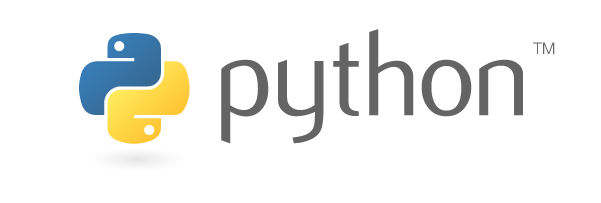